Merge statement in APEX on any sObject
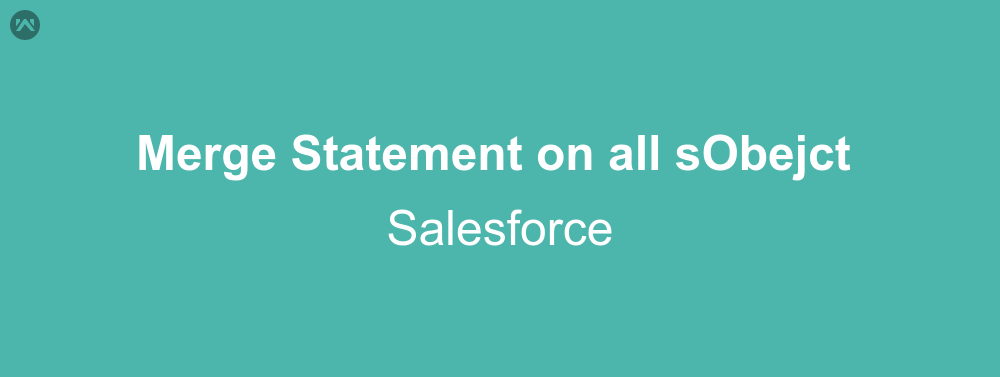
We all know that Apex support various DML statements, like insert, update, delete. One of these statements is Merge statement, which is not very much used due to fact that it can only be used on the sObjects Leads, Accounts and Contacts. Although if we had an option to use this functionality on other objects then a lot of excessive coding can be avoided. So, let’s actually create a function that mimics the functionality of merge statement.
Apex Class
Let’s dive into the apex code for the said problem.
public class MergeClass { public static String MergeObjects(List records){ if(records.size() == 0){ return 'error: Atlease 1 record is required'; } if(records.size() == 1){ return records[0].id; } String sObjectType = records[0].getsObjectType().getDescribe().getName(); Map fields = Schema.getGlobalDescribe().get(sObjectType).getDescribe().fields.getMap(); SavePoint sp = Database.setSavepoint(); try{ for(string str: fields.keySet()){ if(records[0].get(str) == null){ for(integer i = 1; i<records.size(); i++){ if(records[i].get(str) != null){ records[0].put(str, records[i].get(str)); break; } } } } list objects = new list(); for(integer i=1;i<records.size();i++){ objects.add(records[i]); } delete objects; upsert records[0]; return records[0].id; }catch(exception e){ database.rollback(sp); return e.getMessage(); } } }
Here in this class, we have written a static function that takes a list of sObjects and then iterates over them. We have taken sObject as it can be easily manipulated with the help of get() and set() methods, and is a more dynamic approach towards our issue. We have merged all the sObjects of this list into the first sObject. Once the data copy procedure is complete we can delete the sObjects and keep only the sObject at 0 indexes to be upserted. Although if the insert fails all the deleted records will be rolled back and the error message will be returned. Which means that the transaction remains atomic.
Also after this code, the delete trigger of all the other records will be called which is a standard functionality of merge operation. This way we have mimiced all the functionality of merge operation and without taking too many resources, queries or time.
In order to test this code go to the Execute ananymous window and paste the following code there.
list accs = new list(); accs.add(new account(name = 'test1', accountNumber = '1212')); accs.add(new account(name = 'test2', accountNumber = '1111')); accs.add(new account(name = 'test3')); insert accs; system.debug(accs); system.debug(MergeClass.MergeObjects((List)accs));
Support
That’s all about merge statement on all sObjects, for any further queries feel free to contact us at:
https://wedgecommerce.com/contact-us/
Or let us know your views about this blog in the comments section below.