Dynamic Field Mapping In Salesforce
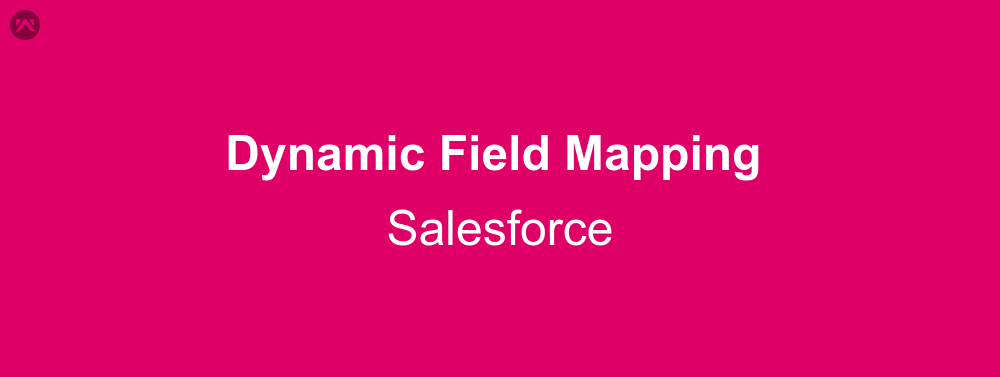
In this blog we are going to learn about dynamic field mapping in salesforce apex class. As a developer you may have faced this challenge to map the field and value while dynamically creating a record. So let’s see an example to create an account in which we map its field to value dynamically.
Example
#Step 1 : Create a apex class ‘dynamicFieldUpdateEg‘ and paste the following code.
public with sharing class dynamicFieldUpdateEg { /** * Webkul Software. * * @category Webkul * @author Webkul * @copyright Copyright (c) 2010-2018 Webkul Software Private Limited (https://webkul.com) * @license https://store.webkul.com/license.html */ public String selectedField {get; set;} public String fieldValue {get; set;} public String accName {get; set;} // Get all fields if string type and which is updateable dynamically by using schema class. public List getAllAccField() { List options = new List(); Map <String, Schema.SObjectField> fieldMap = Schema.getGlobalDescribe().get('Account').getDescribe().fields.getMap(); Map<String, String> preDefinedMap = new Map<String, String>(); for(Schema.SObjectField sfield : fieldMap.Values()){ String strType = string.valueOf(fieldMap.get(sfield.getdescribe().getname()).getDescribe().getType()); if(strType == 'STRING' && sfield.getdescribe().isAccessible() == true && sfield.getdescribe().isUpdateable() == true) { options.add(new SelectOption(sfield.getdescribe().getname(), sfield.getdescribe().getlabel())); } } return options; } public void saveData() { try { Account acc = new Account(); acc.Name = accName; acc.put(selectedField, fieldValue); insert acc ; } catch (Exception ex) { system.debug(ex.getMessage()); } } }
In above example we put selected field with entered value from visualforce page by using following code.
acc.put(selectedField, fieldValue);
#Step2 : Now create a visualforce page as follows :
<apex:page controller="dynamicFieldUpdateEg"> <!-- /** * Webkul Software. * * @category Webkul * @author Webkul * @copyright Copyright (c) 2010-2018 Webkul Software Private Limited (https://webkul.com) * @license https://store.webkul.com/license.html */ --> <apex:form> <table> <tr> <th> Enter Account Name</th> <th> Select Field </th> <th> Enter Value </th> <th> Action </th> </tr> <tr> <td> <apex:inputText value="{!accName}"></apex:inputText> </td> <td> <apex:selectList size="1" value="{!selectedField}"> <apex:selectOptions value="{!AllAccField}"></apex:selectOptions> </apex:selectList> </td> <td> <apex:inputText value="{!fieldValue}"></apex:inputText> </td> <td> <apex:commandButton action = "{!saveData}" value="Save"/> </td> </tr> </table> </apex:form> </apex:page>
Support
That’s all about dynamic field mapping in salesforce apex class, still if you have any further query, feel free to contact us, we will be happy to help you
https://wedgecommerce.com/contact-us/