Display picklist values dynamically in lightning component using apex
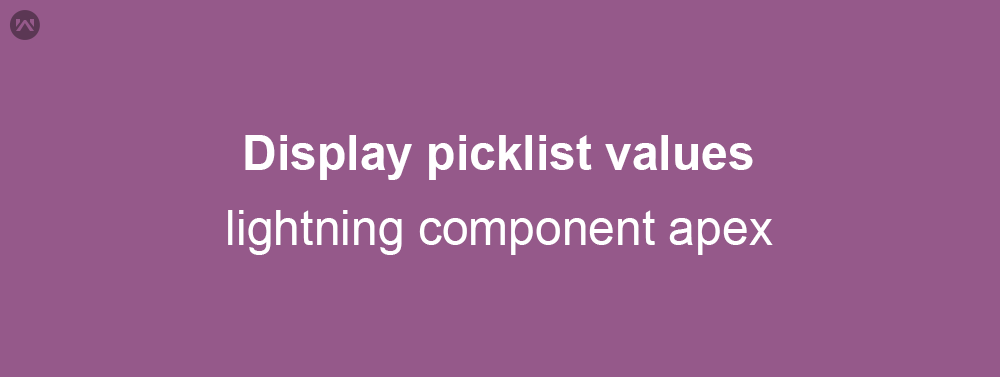
In this blog, we will see how to get picklist values of a field dynamically using apex in lightning component.
In the below given example we will fetch the Industry field value of lead object to display it on the lightning component, and create a lead record.
DynamicPicklist Apex Controller:
public class DynamicPicklist { @AuraEnabled Public static void createLead(Lead leadobj){ try{ insert leadobj; }catch(Exception e){ System.debug(e.getMessage()); System.debug(e.getLineNumber()); } } @AuraEnabled public static Map<String, String> getIndustryFieldValue(){ Map<String, String> options = new Map<String, String>();
Schema.DescribeFieldResult fieldResult = Lead.Industry.getDescribe(); List<Schema.PicklistEntry> pValues = fieldResult.getPicklistValues(); for (Schema.PicklistEntry p: pValues) { options.put(p.getValue(), p.getLabel()); } return options; } }
DynamicPicklistComponent.cmp
<aura:component controller="DynamicPicklist" implements="flexipage:availableForAllPageTypes,force:appHostable"> <aura:attribute name="fieldMap" type="Map"/> <aura:attribute name="lead" type="Lead" default="{'sobjectType':'Lead', 'LastName': '', 'Company': '', 'Email': '', 'Phone': '', 'Industry': ''}"/> <!--Declare Handler--> <aura:handler name="init" value="{!this}" action="{!c.doInit}"/> <!--Component Start--> <div class="slds-m-around--xx-large"> <div class="container-fluid"> <div class="form-group"> <lightning:input name="Name" type="text" label="Lead Name" value="{!v.lead.LastName}" /> </div> <div class="form-group"> <lightning:input name="Company" type="text" label="Company" value="{!v.lead.Company}" /> </div> <div class="form-group"> <lightning:input name="Email" type="email" label="Email" value="{!v.lead.Email}" /> </div> <div class="form-group"> <lightning:input name="Phone" type="phone" label="Phone" value="{!v.lead.Phone}" /> </div> <div class="form-group"> <lightning:select aura:id="industryPicklist" value="{!v.lead.Industry}" onchange="{!c.handleOnChange}" name="industryPicklist" label="Industry" required="true"> <option value="">--None--</option> <aura:iteration items="{!v.fieldMap}" var="i" indexVar="key"> <option text="{!i.value}" value="{!i.key}" selected="{!i.key==v.lead.Industry}" /> </aura:iteration> </lightning:select> </div> </div> <br/> <lightning:button variant="brand" label="Submit" onclick="{!c.LeadSave}" /> </div> </aura:component>
DynamicPicklistComponentController.js
({ doInit: function(component, event, helper) { helper.getPicklistValues(component, event); }, LeadSave : function(component, event, helper) { helper.saveLead(component, event); }, //handle Industry Picklist Selection handleOnChange : function(component, event, helper) { var industry = component.get("v.lead.Industry"); alert(industry); } })
DynamicPicklistComponentHelper.js
({ getPicklistValues: function(component, event) { var action = component.get("c.getIndustryFieldValue"); action.setCallback(this, function(response) { var state = response.getState(); if (state === "SUCCESS") { var result = response.getReturnValue(); var fieldMap = []; for(var key in result){ fieldMap.push({key: key, value: result[key]}); } component.set("v.fieldMap", fieldMap); } }); $A.enqueueAction(action); }, saveLead : function(component, event) { var lead = component.get("v.lead"); var action = component.get("c.createLead"); action.setParams({ leadobj : lead }); action.setCallback(this,function(response){ var state = response.getState(); if(state === "SUCCESS"){ alert('Record Created Successfully!!'); } else if(state === "ERROR"){ var errors = action.getError(); if (errors) { if (errors[0] && errors[0].message) { alert(errors[0].message); } } } }); $A.enqueueAction(action); } })