Calling Apex Controller Method In Lightning Component
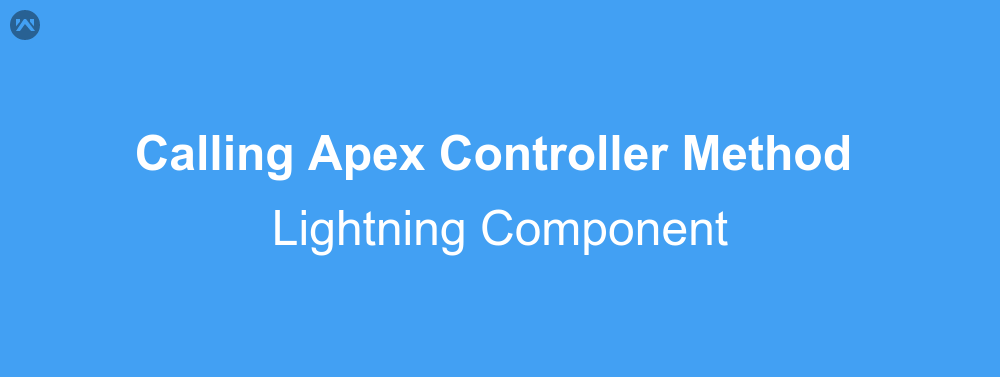
In this blog we are going to learn about calling apex controller method in lightning component. Lightning experience make easier to build web application more efficient and faster. It uses client site controller and LDS (lightning data service) which makes your web application more faster by reducing server round trip. But web application is not bounded to client site tasks. We do need to perform some business login which can be done by using apex controller method only. So let’s learn how to do this.
Lightning Component
Let’s say we need to show the list of Account, contact and lead dynamically. So to perform this tasks we need to define a select list of entity type and trigger a client side action onchange of the entity type. This client side action call server side method which returns list of the selected entity.
To perform this tasks, we need to define apex class in component controller like defined in below code.
<!-- /** * Webkul Software. * * @category Webkul * @author Webkul * @copyright Copyright (c) 2010-2017 Webkul Software Private Limited (https://webkul.com) * @license https://store.webkul.com/license.html */ -- > <!-- define the apex class in component controller attribute -- > <aura:component controller="entityTypeController" > <aura:attribute name='componentString' type='String' default="Account" description='Selected entity type'/ > <aura:attribute name='sObjList' type='sObject[]' description = 'Returned list from server side controller'/ > <aura:handler name='init' value='{!this}' action='{!c.changeEntity}' description = 'Trigger defined action on initialization of component'/ > <div > <!-- Define select list for entity type -- > <lightning:select label='Entity Type' name='cmpString' value='{!v.componentString}' onchange='{!c.changeEntity}' > <option value='Account' >Account </option > <option value='Contact' >Contact </option > <option value='Lead' >Lead </option > </lightning:select > <!-- Show the returned value from server side controller method -- > <table class="slds-table slds-table_bordered slds-table_cell-buffer" > <tr > <th >Id </th > <th >Name </th > </tr > <aura:iteration items='{!v.sObjList}' var="sObj" > <tr > <td >{!sObj.Id} </td > <td >{!sObj.Name} </td > </tr > </aura:iteration > </table > </div > </aura:component >
Client Side Controller
To call the server side method we need to create the instance of server side method in client side controller.
We have two methods to set the parameter of server side method and get the response :
1). setParams() : To set the parameter of server side controller method.
2). setCallback(this, function(response){}) : To perform the tasks when we get the response of server side controller method.
A client-side action could cause multiple events,which could trigger other events and other server-side action calls. So we need to add $A.enqueueAction method to add the server-side action to the queue.
({ /** * Webkul Software. * * @category Webkul * @author Webkul * @copyright Copyright (c) 2010-2017 Webkul Software Private Limited (https://webkul.com) * @license https://store.webkul.com/license.html */ changeEntity : function(component, event, helper) { var action = component.get('c.getEntity'); // method name i.e. getEntity should be same as defined in apex class // params name i.e. entityType should be same as defined in getEntity method action.setParams({ "entityType" : component.get('v.componentString') }); action.setCallback(this, function(a){ var state = a.getState(); // get the response state if(state == 'SUCCESS') { component.set('v.sObjList', a.getReturnValue()); } }); $A.enqueueAction(action); } })
Server Side Controller
Now define @AuraEnabled method in server side controller to expose the method to client side.
For @AuraEnabled method there is two necessary condition:
– It should be public or global
– It should be static
public class entityTypeController { /** * Webkul Software. * * @category Webkul * @author Webkul * @copyright Copyright (c) 2010-2017 Webkul Software Private Limited (https://webkul.com) * @license https://store.webkul.com/license.html */ @AuraEnabled public static List getEntity(String entityType){ List sobj = new List(); if(entityType != null) { String query = 'SELECT Name, Id FROM '+entityType ; sobj = database.query(query); } return sobj; } }
Support
That’s all for calling apex controller method in lightning component. , still if you have any further query or seek assistance to make your salesforce classic apps compatible with lightning experience, feel free to contact us, we will be happy to help you https://wedgecommerce.com/contact-us/.